Command Console
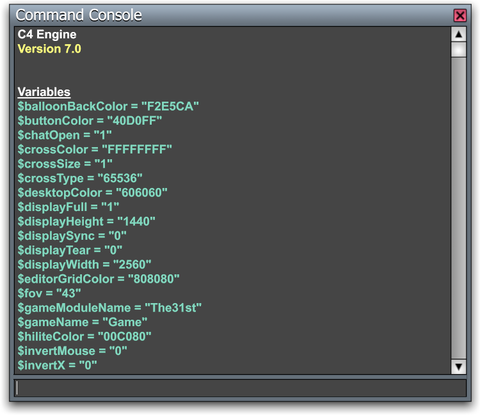
The Command Console window can be opened at any time by pressing the tilde/grave key. (On some non-English keyboards, the key directly below the Escape key should be used.) The window displays a command line and an output buffer as shown in the image to the right. If the C4 Engine was built using the debug settings, the window displays "(Debug)" to the right of the build number.
The console stores a small command history that can be accessed with the up and down arrow keys.
Core Engine Commands
The following table describes some of the commands that are built into the engine. New commands can be added by an application or plugin module (see below).
Command |
Description |
|
Displays the IP address of the local machine. Must be in a network game. |
|
Binds the key whose name is key to the command specified by command. Whenever the bound key is pressed in gameplay mode, the specified command is executed. |
|
Toggles the display of bounding boxes for active rigid bodies. |
|
Displays a list of available console commands. |
|
Disconnects from a multiplayer game. |
|
Writes a list of all currently allocated memory blocks to the file |
|
Executes the file |
|
Opens the Texture Generation tool, which renders textures for light projections, environment maps, and impostor textures. (Can only be invoked while a game world is running.) |
|
(Advanced) Displays memory heap totals in the console. |
|
Loads the world |
|
(Advanced) Toggles the display of light regions. |
|
Opens the Network window, which displays various network statistics. (The Network window can also be opened by choosing C4 > Network Window.) |
|
Toggles the display of normal vectors. |
|
Quits the C4 Engine. |
|
Starts recording video and/or audio to files that can later be imported as a movie. See Recording Movies for more information. |
|
Resolves a host name to an IP address. Must be in a network game. |
|
Restore the previously saved game |
|
Save the current game as |
|
Sends a chat message to all players in the current multiplayer game. |
|
Takes a screenshot and saves it as |
|
(Advanced) Opens the Shadow Map window for a particular light type. The value of type must be |
|
(Advanced) Toggles the display of sound flow paths. |
|
Opens the Stats window, which displays various engine statistics. (The Stats window can also be accessed by choosing C4 > Stats Window.) |
|
Toggles the display of tangent vectors. |
|
Opens the Time window, which displays the frame rate in frames/s and ms/frame, the current load on the GPU and CPU, and a detailed breakdown of the time the engine spends on specific features such as shadows and post processing. (The Time window can also be opened by choosing C4 > Time Window.) |
|
Unbinds the key whose name is key. |
|
Undefines the variable name, if the variable is not permanent. |
|
Unloads the current gameplay world. |
|
Displays a list of currently defined system variables. |
|
Toggles the display of the wireframe overlay. |
Tool Commands
The following commands are defined by the standard tools that ship with the C4 Engine. Most of these commands have equivalent items in the C4 Menu. If the name parameter is omitted from any of these commands, then a file picker dialog will appear to let you select a file.
Command |
Description |
|
Opens the Font Importer tool. (This tool can also be opened by choosing C4 > Import Font.) |
|
Opens the Movie Importer tool. (Movies can also be imported by choosing C4 > Import Movie.) |
|
Imports the sound file |
|
Imports the string table file |
|
Imports the texture file |
|
Opens the model file |
|
Opens the movie file |
|
Creates a pack file, where name is the name of a top-level subfolder in the |
|
Opens the panel file |
|
Opens the sounds file |
|
Opens the texture file |
|
Opens the world file |
Defining New Commands
Application modules and plugin modules can add their own commands to the engine by creating new instances of the Command
class. The constructor for the Command
class takes a command string and a pointer to an observer object that is invoked when the command is executed. For example, to define a command called explode that causes the MyClass::ExplodeFunction()
function to be called, you would construct a new Command
instance as follows.
CommandObserver<MyClass> explodeObserver(this, &MyClass::ExplodeFunction);
Command *explodeCommand = new Command("explode", &explodeObserver);
Once the command has been constructed, it needs to be added to the engine's command list before it will be recognized. This is done by calling the Engine::AddCommand()
function. Continuing the explode example, you would make the following call to add the explode command to the engine.
TheEngine->AddCommand(explodeCommand);
Destroying the Command
instance automatically removes the command from the engine.
The observer event handling function assigned to a new command must have the following prototype.
void ExplodeFunction(Command *command, const char *text);
When a command is entered into the console (or executed from a file), the observer event handler is invoked, and the text passed in through the text parameter provides the rest of the command line. For instance, the command explode 1 boom
would cause the ExplodeFunction()
function to be called with a pointer to the string "1 boom"
.
Console Output
You can write text to the Command Console window by calling the Engine::Report()
function. The string passed to the Engine::Report()
function is output to the console whenever the flags
parameter does not include the bit kReportLog
.
For example, to write some integer value to the console with a text label, you could make the following call:
Engine::Report(String<63>("My value is now ") += myValue);
(The number 63 represents the maximum length of the string stored in the String
object. This number can be omitted altogether to get an arbitrarily large string, but it causes extra memory allocations.)
Disabling the Console
The Command Console window can be opened when the keyboard is in either interface mode or game input mode, and both of these methods must be disabled in order to prevent access to the console. To make the Command Console window completely inaccessible, make the following two function calls:
TheInputMgr->SetConsoleCallback(nullptr);
TheInterfaceMgr->DisableConsole();
If the Command Console window is later going to be enabled again, then it will also be necessary to save the previous console procedure so that it can be restored. This can be done by expanding the above code as follows:
// Declared in some larger scope:
InputMgr::KeyCallback savedConsoleCallback;
void *savedConsoleCookie;
...
savedConsoleCallback = TheInputMgr->GetConsoleCallback();
savedConsoleCookie = TheInputMgr->GetConsoleCookie();
TheInputMgr->SetConsoleCallback(nullptr);
TheInterfaceMgr->DisableConsole();
To enable the Command Console window again and restore the previous console procedure in the Input Manager, use the following code:
TheInputMgr->SetConsoleProc(savedConsoleProc, savedConsoleCookie);
TheInterfaceMgr->EnableConsole();